How Redis Helps to Increase Service Performance in NodeJS
In modern backend applications, performance optimization is crucial for handling high traffic efficiently. Typically, a backend application consists of business logic and a database. Initially, developers focus on implementing functionality, often overlooking performance considerations. As traffic increases, database operations become a bottleneck, leading to high server loads. System administrators often respond by scaling server configurations to support the growing load. However, can we handle the same load on a lower configuration server? The answer is yes, provided the code is optimized effectively. One of the most powerful solutions for optimizing backend performance is Redis caching.
What is Redis?
Redis (Remote Dictionary Server) is an open-source, in-memory data structure store that can be used as a database, cache, or message broker. Due to its in-memory nature, Redis is incredibly fast compared to traditional databases. It supports various data structures like strings, lists, sets, sorted sets, and hashes, making it a versatile tool for performance optimization.
How Redis Helps
1. Redis as a Caching Solution
One of the most common uses of Redis is as a caching layer to reduce frequent database queries. Instead of querying the database for every request, we can store frequently accessed data in Redis and retrieve it directly, significantly reducing database load.
Example: Caching User Data
Consider a scenario where we frequently fetch user data from a database. Instead of querying the database every time, we can first check Redis. If the data is available in Redis, we return it immediately; otherwise, we fetch from the database and store it in Redis for future use.
const redis = require('redis');
const client = redis.createClient();
const db = require('./database'); // Assume this is a database module
async function getUser(userId) {
return new Promise((resolve, reject) => {
client.get(`user:${userId}`, async (err, data) => {
if (err) reject(err);
if (data) {
console.log('Cache hit');
return resolve(JSON.parse(data));
}
console.log('Cache miss');
const user = await db.getUserById(userId);
client.set(`user:${userId}`, JSON.stringify(user), {EX: 60*60}); // Store in Redis and Add expiry for 1 hour
resolve(user);
});
});
}
2. Automatic Expiry of Data
Redis provides an expiration mechanism to automatically delete stale data after a specified period. This ensures that the cache does not store outdated data indefinitely.
client.set('session:12345', 'user_data', {EX: 60*30}); // Expires after 30 minutes
3. Redis in Microservice Architectures (Pub/Sub)
In microservice architectures, multiple services often need to communicate with each other. Instead of making multiple API calls to send the same data to different services, Redis offers a publish-subscribe (Pub/Sub) mechanism where services subscribe to a channel and receive messages when an event is published.
Example: Broadcasting Events to Multiple Services
Consider a scenario where a payment service needs to notify multiple services (e.g., order service, notification service) when a payment is completed. Instead of making multiple API calls, the payment service can publish an event, and all interested services will receive it.
Publishing an Event:
const redis = require('redis');
const publisher = redis.createClient();
function publishPaymentEvent(orderId) {
const event = { orderId, status: 'completed' };
publisher.publish('payment_channel', JSON.stringify(event));
}
Subscribing to an Event:
// Variables
const host = '';
const post = '';
const password = '';
const options = {
url: `redis://${host}:${port}`,
password: `${password}`, // remove if redis does not need password
};
const client = createClient(options);
const subscriber = client.duplicate();
client.on('connect', () => {
logger.info(`[[REDIS]] Redis connected at host ${host} port ${port}`);
});
client.on('error', (err) => {
logger.fatal(`[[REDIS]] Redis Client Error, ${err}`);
});
export const createRedisConnection = async () => {
await client.connect()
await subscriber.connect()
// subscribers
await subscriber.subscribe('payment_channel', ( message) => {
console.log(`Received event on ${message}`);
const event = JSON.parse(message);
// Process the event (e.g., update order status, send notifications)
})
return;
}
4. Session Management Using Redis
Redis is widely used for managing user sessions in web applications. Since it operates in memory, it provides faster read and write operations compared to databases.
Example: Storing Session Data
client.setex(`session:${sessionId}`, 3600, JSON.stringify(sessionData));
Example: Retrieving Session Data
client.get(`session:${sessionId}`, (err, session) => {
if (session) {
console.log('Session data:', JSON.parse(session));
} else {
console.log('Session expired or not found');
}
});
5. Rate Limiting with Redis
Redis can be used for rate limiting to prevent abuse of APIs. By tracking the number of requests a user makes within a time window, we can limit excessive API calls.
Example: Implementing Rate Limiting
const limitRequests = async (userId) => {
return new Promise((resolve, reject) => {
const key = `rate_limit:${userId}`;
client.incr(key, (err, count) => {
if (err) reject(err);
if (count === 1) {
client.expire(key, 60); // Set expiry to 60 seconds
}
if (count > 5) {
resolve(false); // Limit exceeded
} else {
resolve(true);
}
});
});
};
// Middleware to check rate limit
app.use(async (req, res, next) => {
const allowed = await limitRequests(req.user.id);
if (!allowed) return res.status(429).send('Too many requests');
next();
});
Conclusion
Redis is a powerful tool for improving backend performance in multiple ways:
- Caching: Reduces database calls by storing frequently accessed data.
- Expiration Mechanism: Automatically removes stale data.
- Pub/Sub Messaging: Enables event-driven communication between microservices.
- Session Management: Speeds up user authentication and session handling.
- Rate Limiting: Prevents API abuse and ensures fair usage.
By integrating Redis into your backend system, you can handle higher traffic efficiently while reducing the load on your database and servers. If your application faces performance bottlenecks due to frequent database queries, consider Redis as a solution for scalability and efficiency.
Releted Posts
MongoDB 8.0 Performance is 36% higher, but there is a catch…
TLDR: If your app is performance critical, think twice, thrice before upgrading to MongoDB 7.0 and 8.0. Here is why…
Read more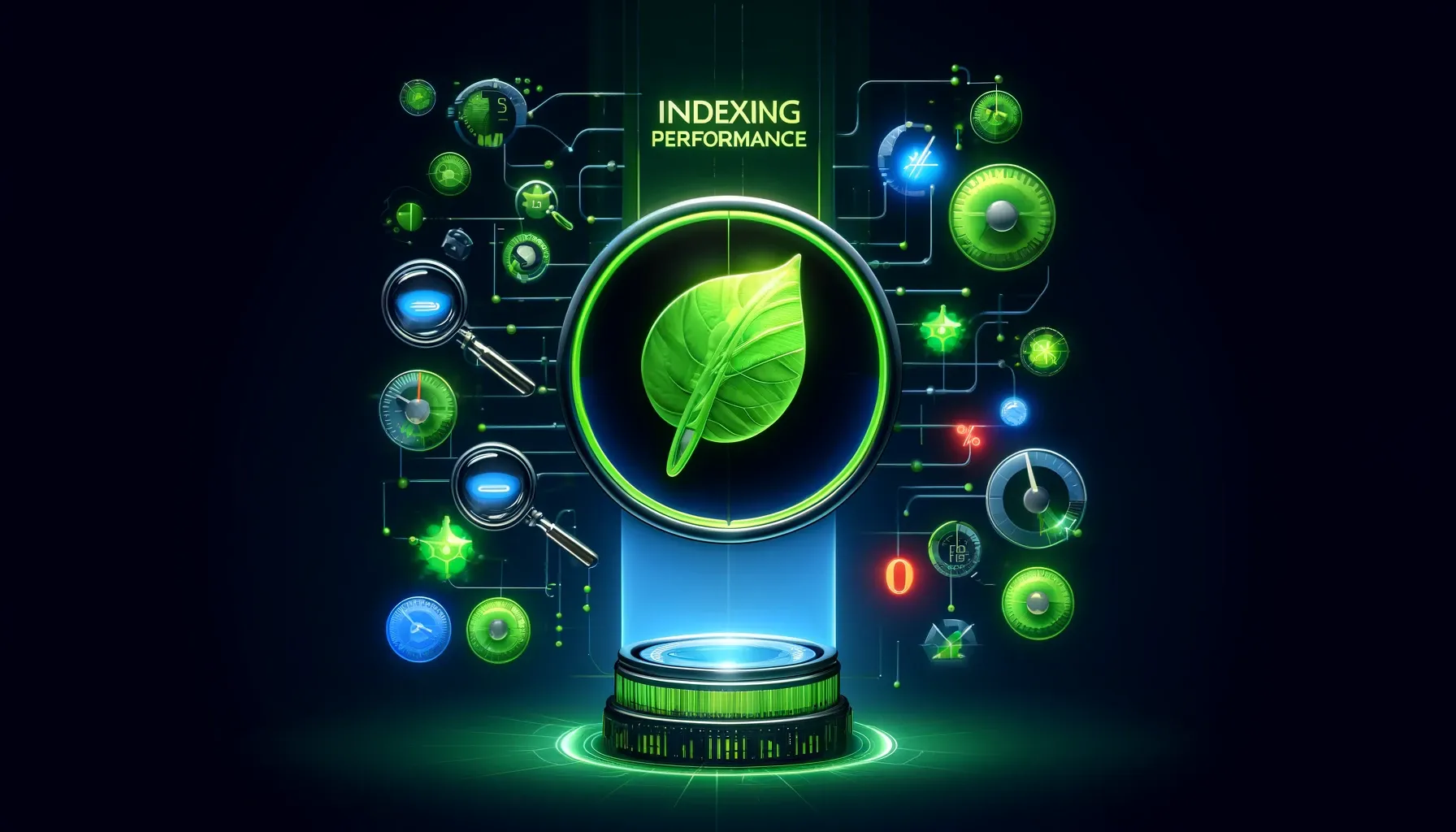
Optimizing MongoDB Performance with Indexing Strategies
MongoDB is a popular NoSQL database that efficiently handles large volumes of unstructured data. However, as datasets grow, query performance can degrade due to increased document scans.
Read moreMongoDB Decay Over Time: How Deletes and Updates Clog Your Database
MongoDB is widely recognized for its flexibility, scalability, and ease of use. However, like many databases, MongoDB’s performance can deteriorate over time, especially in environments with frequent delete and update operations.
Read more